visualControl()v4.0.292
Preview feature: A few issues are known, and filed under this issue.
Creates a control in the right sidebar of the Remotion Studio that allows you to change a value.
Useful for if you have a hardcoded constant that you want to quickly find the optimal value for.
Example
Consider you have a value rotation
that you want to find the optimal value for:
A simple compositiontsx
import {useVideoConfig } from 'remotion';constMyComp :React .FC = () => {constrotation = 180;return <div style ={{rotate : `${rotation }deg`}}>Hello</div >;};
Instead of changing the value of rotation
in the code, you can create a control in the right sidebar of the Remotion Studio that allows you to change the value:
Creating a visual control with the name 'rotation'tsx
import {visualControl } from '@remotion/studio';constMyComp :React .FC = () => {constrotation =visualControl ('rotation', 180);return <div style ={{rotate : `${rotation }deg`}}>Hello</div >;};
Now, in the right sidebar of the Remotion Studio, you will see a control with the name rotation
and a slider to change the value.
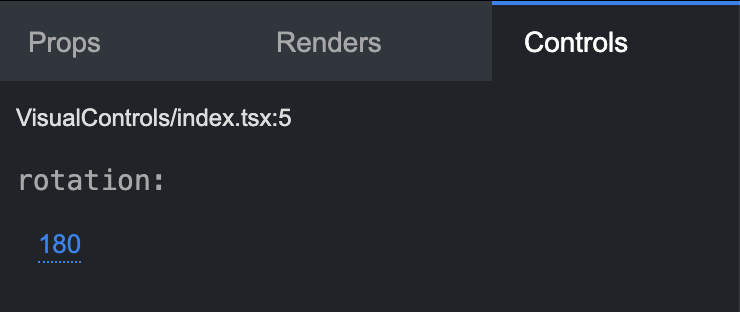
Now you can change the value as you please, until you have found the optimal value.
Once you are happy with the value, you can save it back to the code by clicking the save button in the right sidebar.
Defining a schema
Only primitive values (string
and number
) automatically infer the type of the control.
If you want to define a more complex schema, you can do so by passing a Zod schema as the third argument.
Editing an objecttsx
import {z } from 'zod';constdata =visualControl ('my-data',{rotation : 180,text : 'Hello',},z .object ({rotation :z .number (),text :z .string (),}),);
See: Visual Editing
Editing a colortsx
import {visualControl } from '@remotion/studio';import {zColor } from '@remotion/zod-types';constcolor =visualControl ('my-color', '#fff',zColor ());
See: zColor()
Editing a matrixtsx
import {visualControl } from '@remotion/studio';import {zMatrix } from '@remotion/zod-types';constmatrix =visualControl ('my-matrix', [1, 2, 3, 4],zMatrix ());
See: zMatrix()
When to use
- If you want to create configuration options, use the Props Editor.
- If you want to quickly find the optimal value for a hardcoded constant, use
visualControl()
.
Important to know
The saving feature works only if the first argument of thevisualControl()
function is static, because static analysis is performed on the source file. The following will not work:
❌ This is not possibletsx
// ❌ Not possible to use string interpolationconstname = 'my-data';constdata =visualControl (`rotation-${name }`, 180);// ❌ Not possible to use a variableconstidOutside = 'rotation-my-data';constdataOutside =visualControl (idOutside , 180);